Walkthrough Guides
Rapid and collaborative medical data annotation.
RedBrick AI is a purpose-built SaaS application created to help healthcare AI teams annotate medical data more effectively. RedBrick AI offers web-based annotation tools for CTs, MRIs, X-rays, etc., as well as comprehensive project management and quality control tools.
Request a free trial or product demonstration by contacting us through our website: https://redbrickai.com.
Getting started with guides
Our documentation offers a comprehensive overview of the features and functionality of RedBrick AI. Before diving deep into the documentation, working through our use-case-driven guides to discover RedBrick AI may be useful.
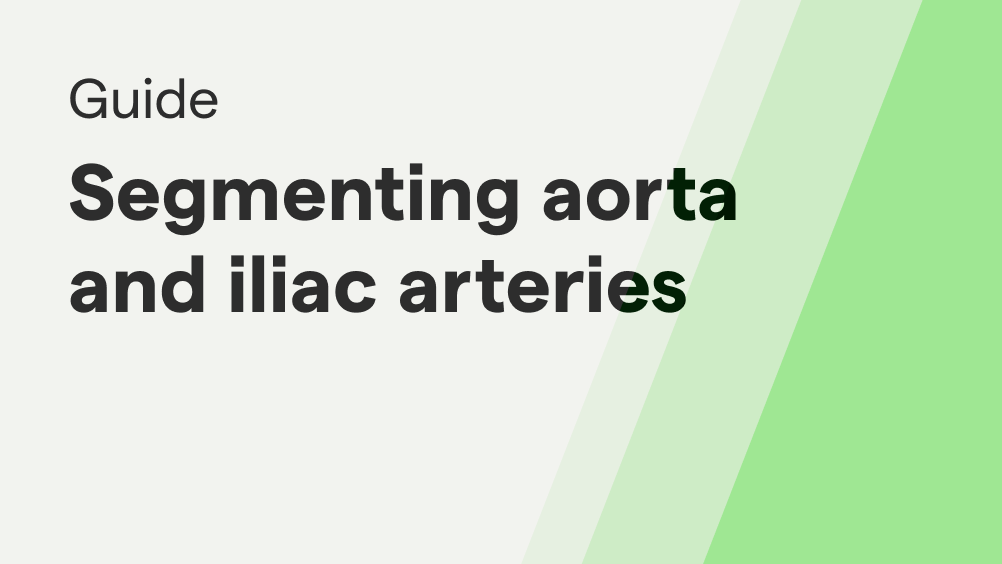
This guide showcases how to use RedBrick AI to segment the aorta and iliac arteries in Abdominal Computed Tomography Angiography scans.
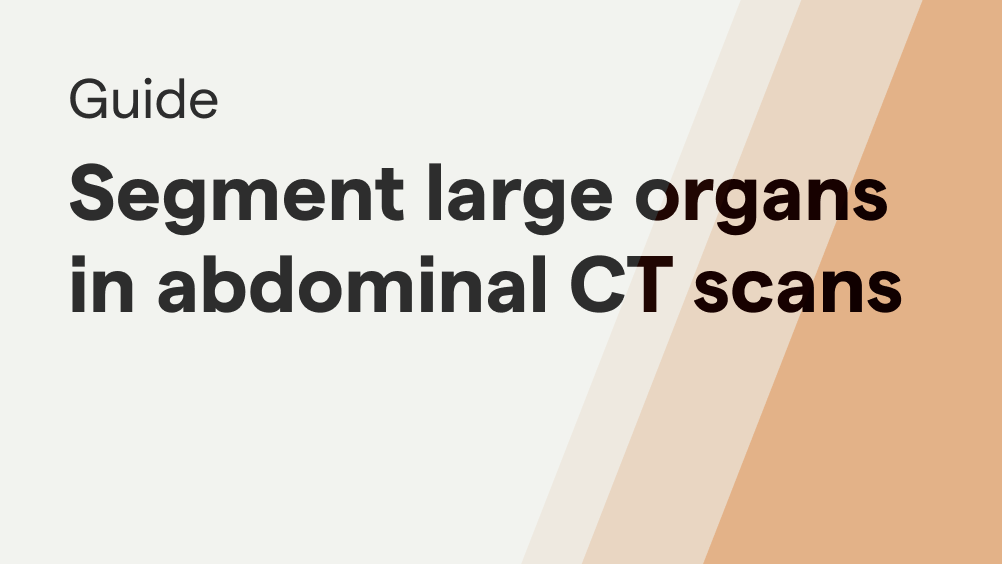
This guide showcases how to use advanced segmentation tools to segment organs like the liver and kidneys in a abdominal CT scan.
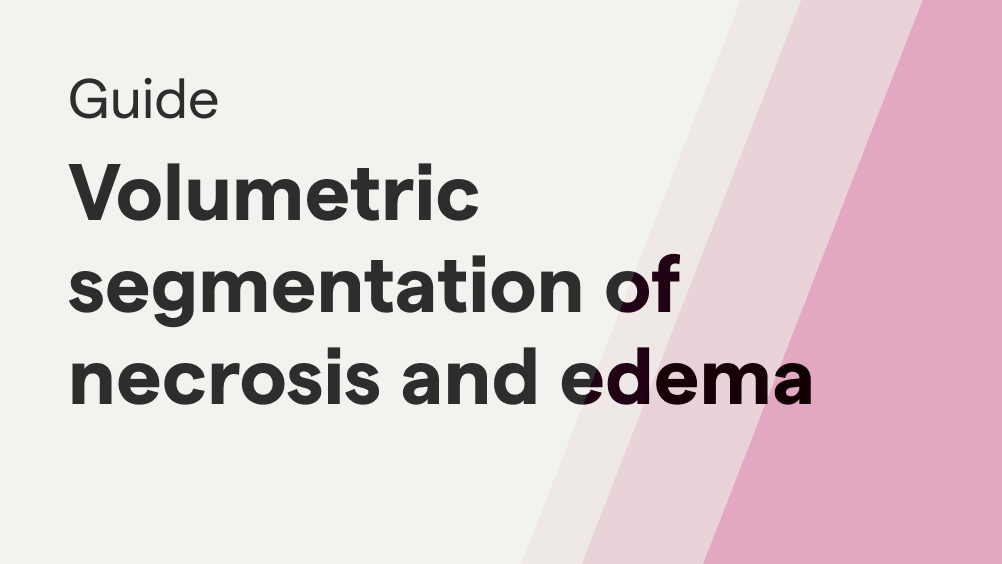
This guide provides a step-by-step overview of configuring your viewer for a 3D MRI study and performing segmentation of necrosis and edema.
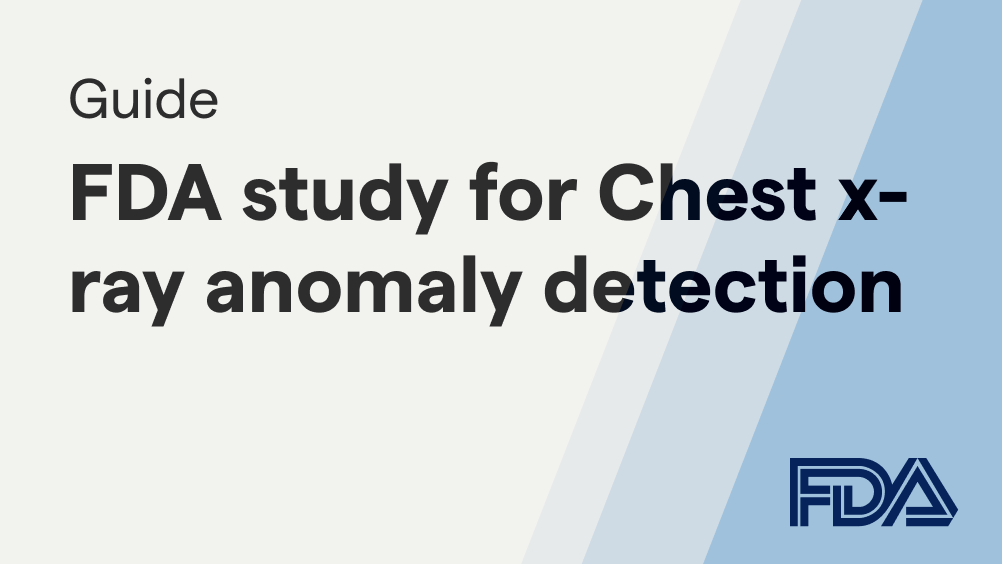
Using RedBrick AI for FDA clinical validation studies. In this guide we walk through a hypothetical study for a chest anomaly algorithm.
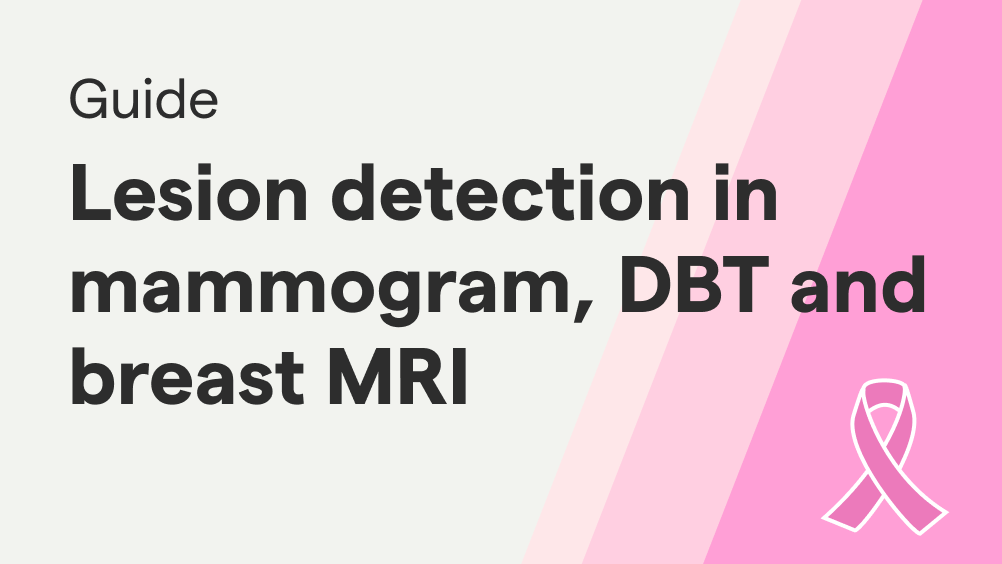
In this guide we will focus on RedBrick AI’s features for breast imaging. We’ll walk through three projects focusing on different modalities, and the special features the platform has for efficient viewing and annotation.